Usually when we develop an application we can replicate the environment where we are running the application to verify that the application works correctly.
Sometimes this is not possible due to some special circumstances (network, special hardware, amount of data, client restrictions,ect..) when we find an error while in this special circumstances, we need to do a remote debug.
Launch the application on the server
First we need to run the application on the server. We can generate a jar file using this instructions.
We need to launch the application with some special arguments:
java -Xdebug -Xrunjdwp:transport=dt_socket,address=1088,server=y,suspend=n -jar hello.jar
Connect Eclipse to the application to debug
To connect to the remote process we need to indicate the host where to connect and the port.
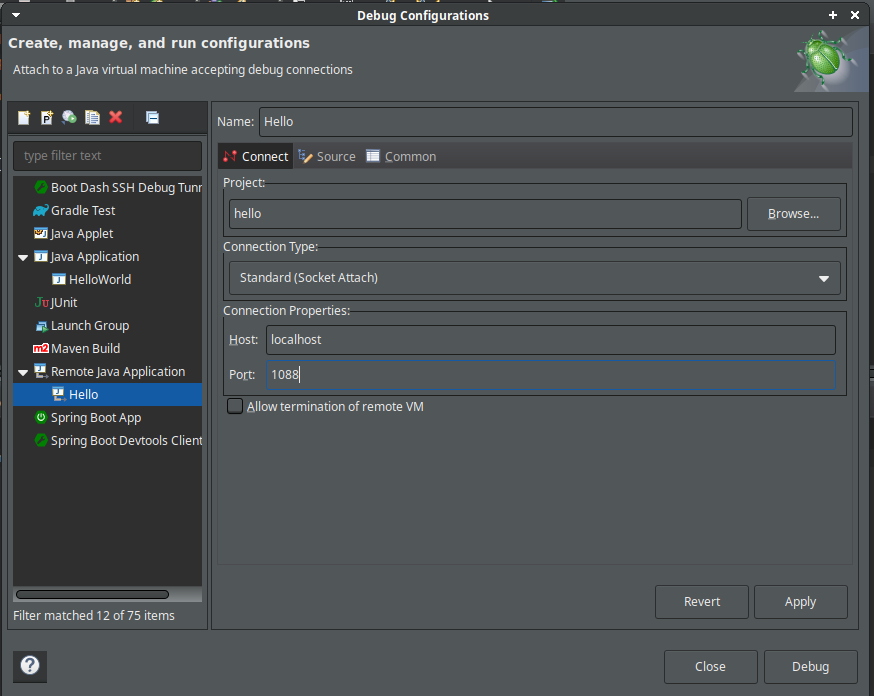
After this, you can debug the application as it was on your local machine.
If you can’t open any port of the server and you only have ssh access this article can be useful