Sentry is another service you don’t know that you need it until you try it.Basically Sentry is a service that catches the exceptions in your code and sends it to a web service to be analyzed, also sends you an email to notify you.
Sentry server is an opensource server that can be installed on your own server, also sentry can work as a SaS and you can use it at https://sentry.io/welcome/
Sentry can work with multiple languages from Python, to JS or Go, you can check all the supported languages here https://sentry.io/platforms/
Configuring sentry with python
First of all you need to create a project like this:
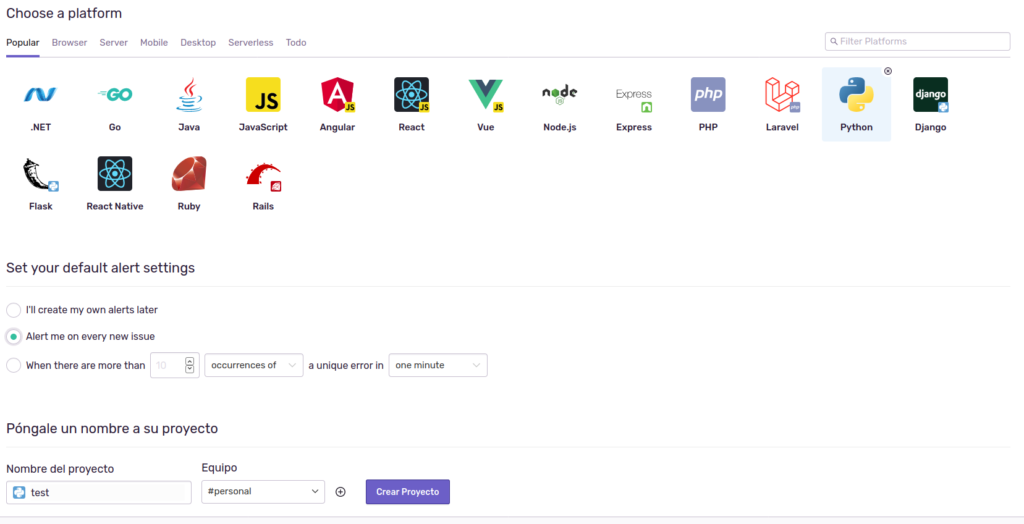
Once you created the project you will get an screen like this
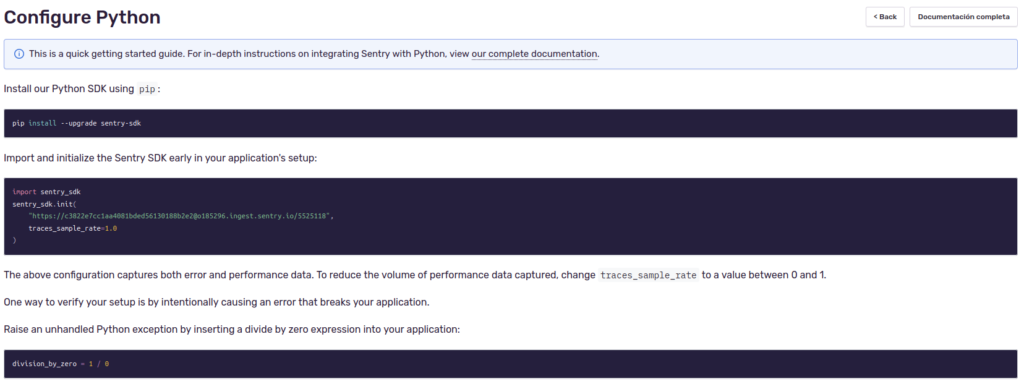
After this you will need to install the sentry package with this command:
pip install --upgrade sentry-sdk
You will need to add this on the package, if you use a setyp.py add it on the install_requires key or if you use it on a requirements.txt file you need to add it.
Now we need to initialize the sentry code. We do it adding this code on the entry point of the code.
import sentry_sdk
sentry_sdk.init(
"!Place here your project DSN"
traces_sample_rate=1.0
)
With this we have our script configured to catch all the exceptions with Sentry.
Extra usage
We can make our code notify an exception manually with sentry with this code:
from sentry_sdk import capture_exception
try:
a=1/0
catch Exception as e:
capture_exception(e)
Also can notify suspicious data without sending an exception with this code:
from sentry_sdk import capture_message
capture_message("Somthing strange")
Deixa un comentari