Sentry is a great tool tool to track errors in our applications, when an error occurs, it is sent to the server, storing it’s trackback and you will receive a notification email. In this article we will learn how to configure sentry on an Ionic Angular Application. On other articles we have seen how to use it with Python.
To explain this, we will start by creating a simple Ionic Application
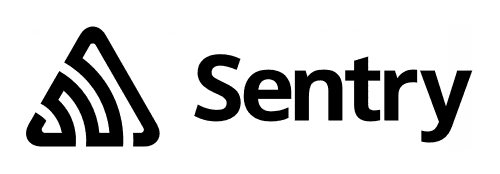
First we create the example application:
ionic start example --type angular tags
Configuration
After this we need to install the npm package
npm install --save @sentry/angular @sentry/tracing
On the main.ts we configure the sentry DSN like this:
import { enableProdMode } from '@angular/core';
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { AppModule } from './app/app.module';
import { environment } from './environments/environment';
import * as Sentry from '@sentry/angular';
import { Integrations } from '@sentry/tracing';
Sentry.init({
dsn: 'your sentry dsn',
integrations: [
new Integrations.BrowserTracing({
tracingOrigins: ['localhost'],
routingInstrumentation: Sentry.routingInstrumentation,
}),
],
// We recommend adjusting this value in production, or using tracesSampler
// for finer control
tracesSampleRate: 1.0,
});
if (environment.production) {
enableProdMode();
}
platformBrowserDynamic()
.bootstrapModule(AppModule)
.catch(err => console.error(err));
Add the ErrorHandler on the providers, on app.module.ts
{
provide: ErrorHandler,
useValue: Sentry.createErrorHandler({
showDialog: false, //You can enable/dissable the report dialog
}),
},
{
provide: Sentry.TraceService,
deps: [Router],
},
{
provide: APP_INITIALIZER,
useFactory: () => () => {},
deps: [Sentry.TraceService],
multi: true,
},
Now, with this your application, it is ready to report to the Sentry server. You can test it with calling an undefined function:
undefinedFunction();
If you want to check extra information about this you can check the official documentation
Deixa un comentari